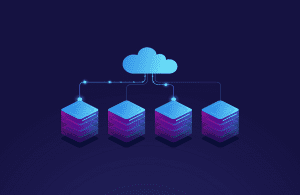
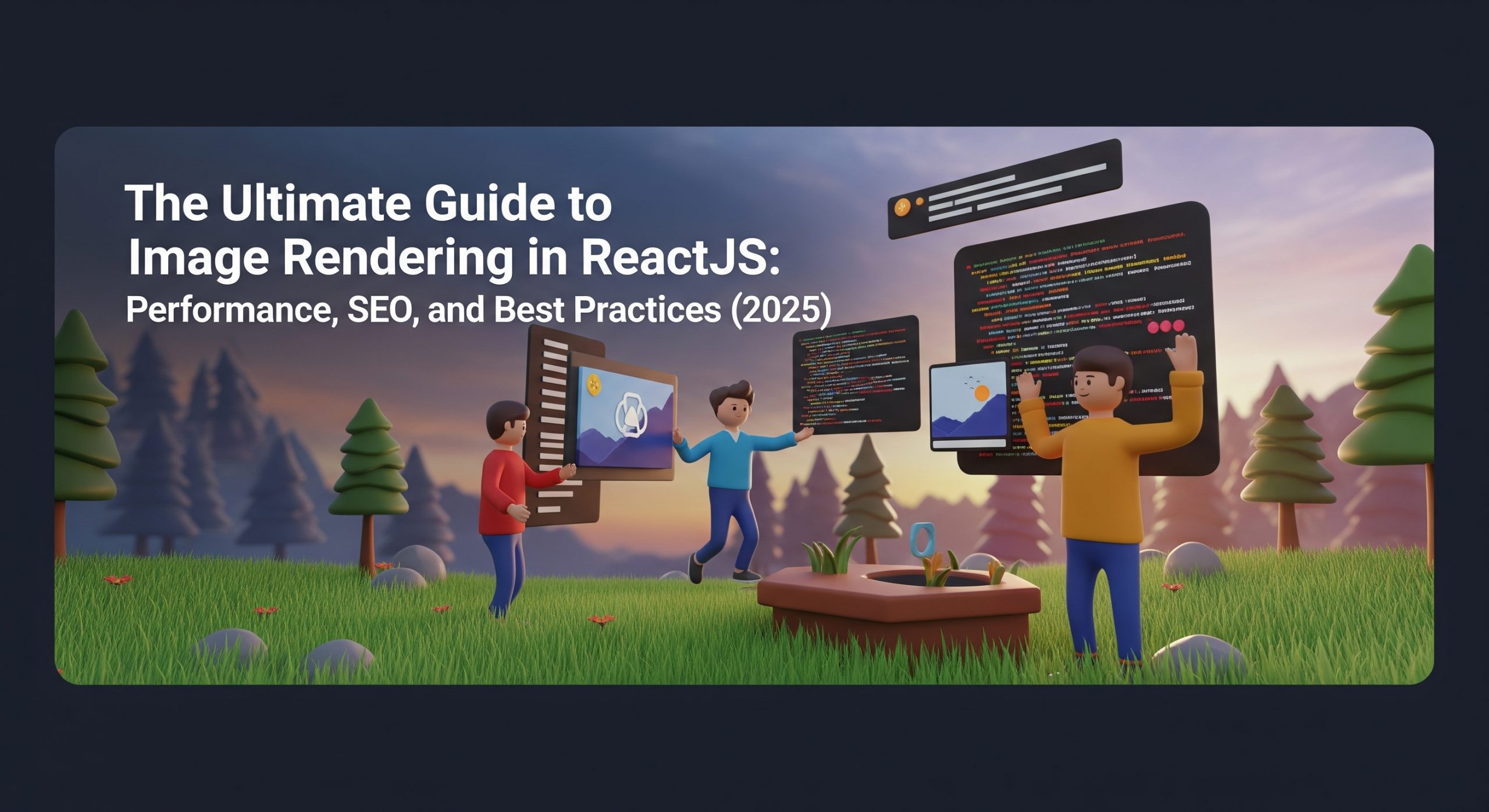
The Ultimate Guide to Image Rendering in ReactJS: Performance, SEO, and Best Practices (2025)
Overview: The Critical Role of Images in Modern Web Development Images are fundamental to web experiences, accounting for approximately 50% of a typical webpage’s total size. How you choose to render these assets significantly impacts user experience, page performance, and search engine rankings. As web technologies evolve, developers have multiple options for implementing images in […]
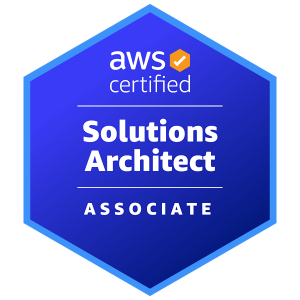
Explore best practices, tutorials, case studies, and insights on leveraging AWS’s vast ecosystem to build, deploy, and manage applications in the cloud
The Design Pattern category explores reusable solutions to common software design challenges, helping developers write efficient, maintainable, and scalable code
The Security category focuses on best practices, tools, and frameworks essential for protecting applications, data, and infrastructure in an increasingly digital world
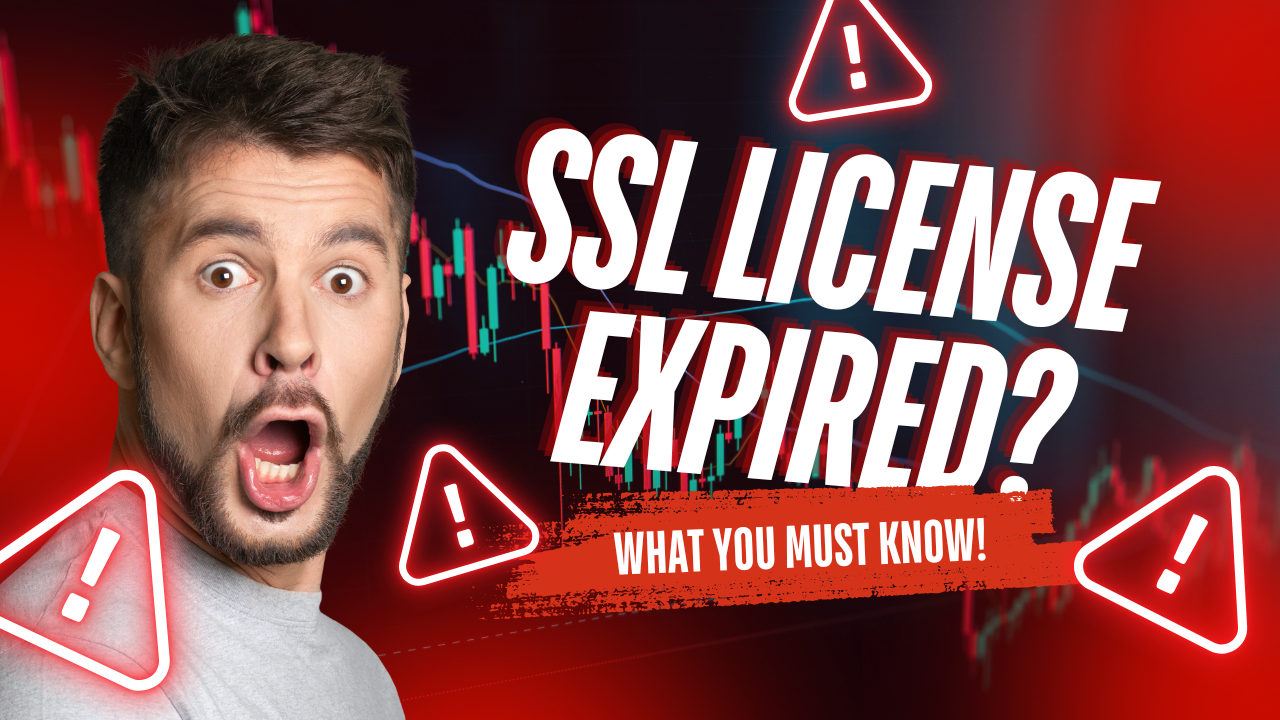
Ultimate Guide to Renewing SSL Certificates: Secure Your Website in 2024
Ensure your website stays secure! 🔒 Learn how to check, renew, and manage your SSL certificate to prevent security risks and downtime. Follow our step-by-step guide with best practices to keep your HTTPS protection active in 2024!
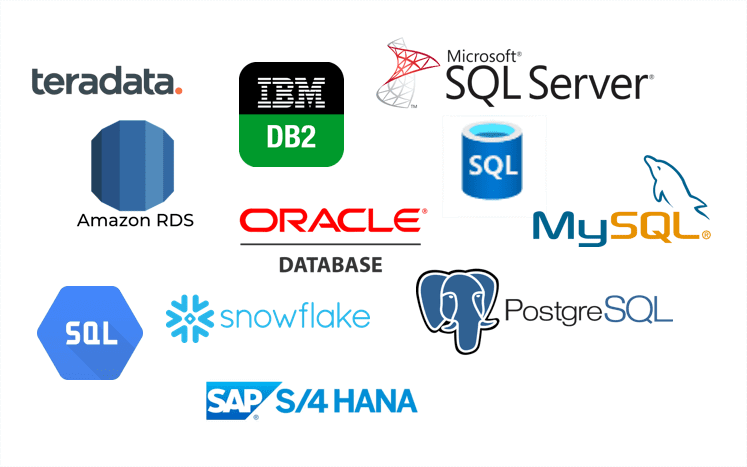
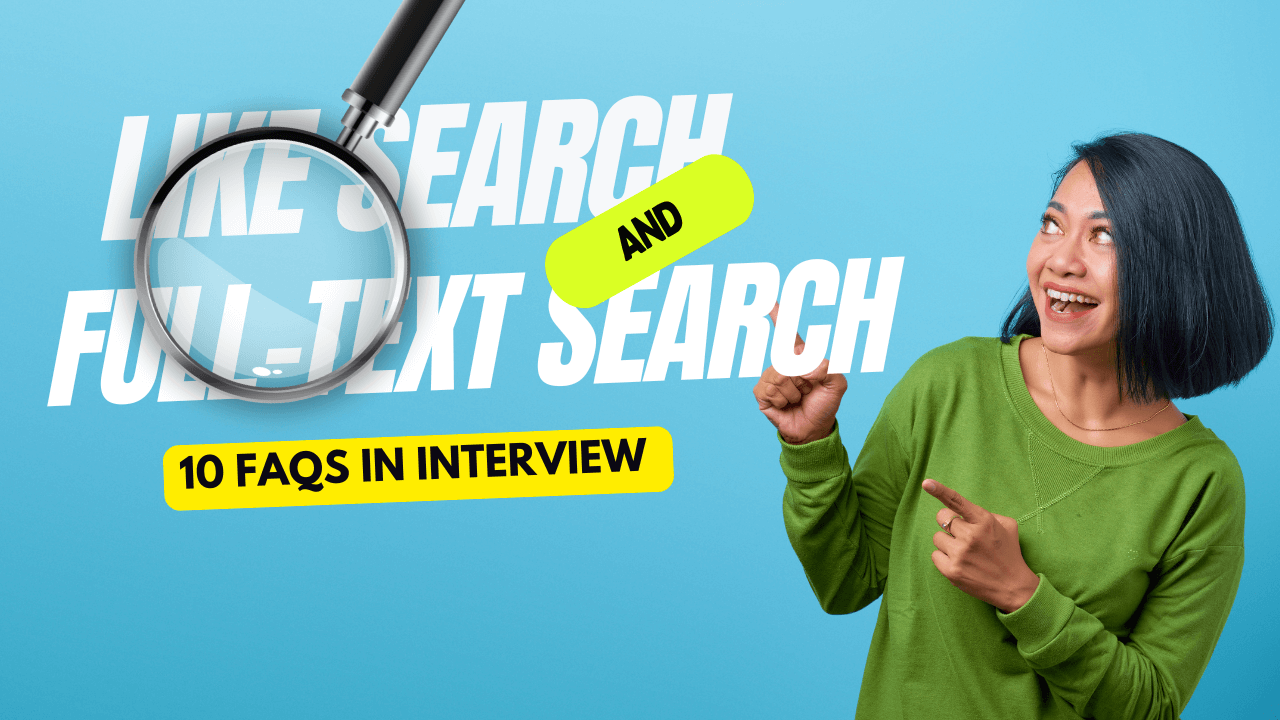
LIKE vs Full-Text Search: SQL Performance and Use Cases
Explore the differences between SQL’s LIKE operator and Full-Text Search. Learn their syntax, performance, use cases, and advanced features for optimizing database queries
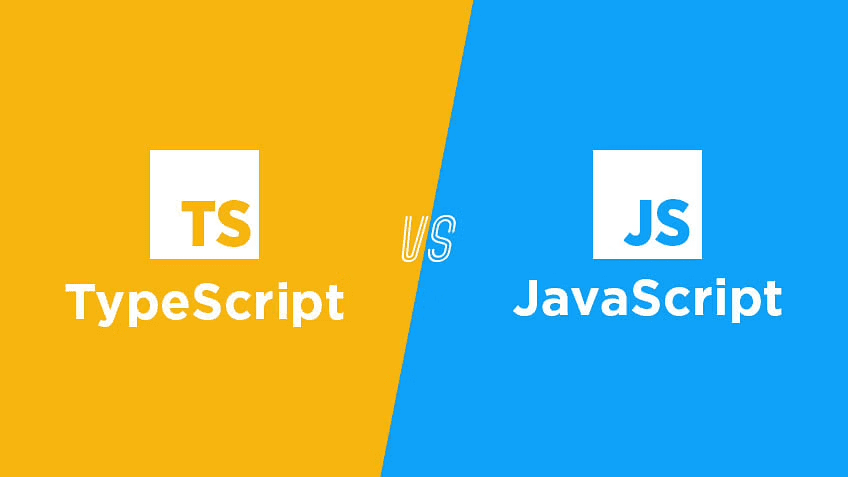
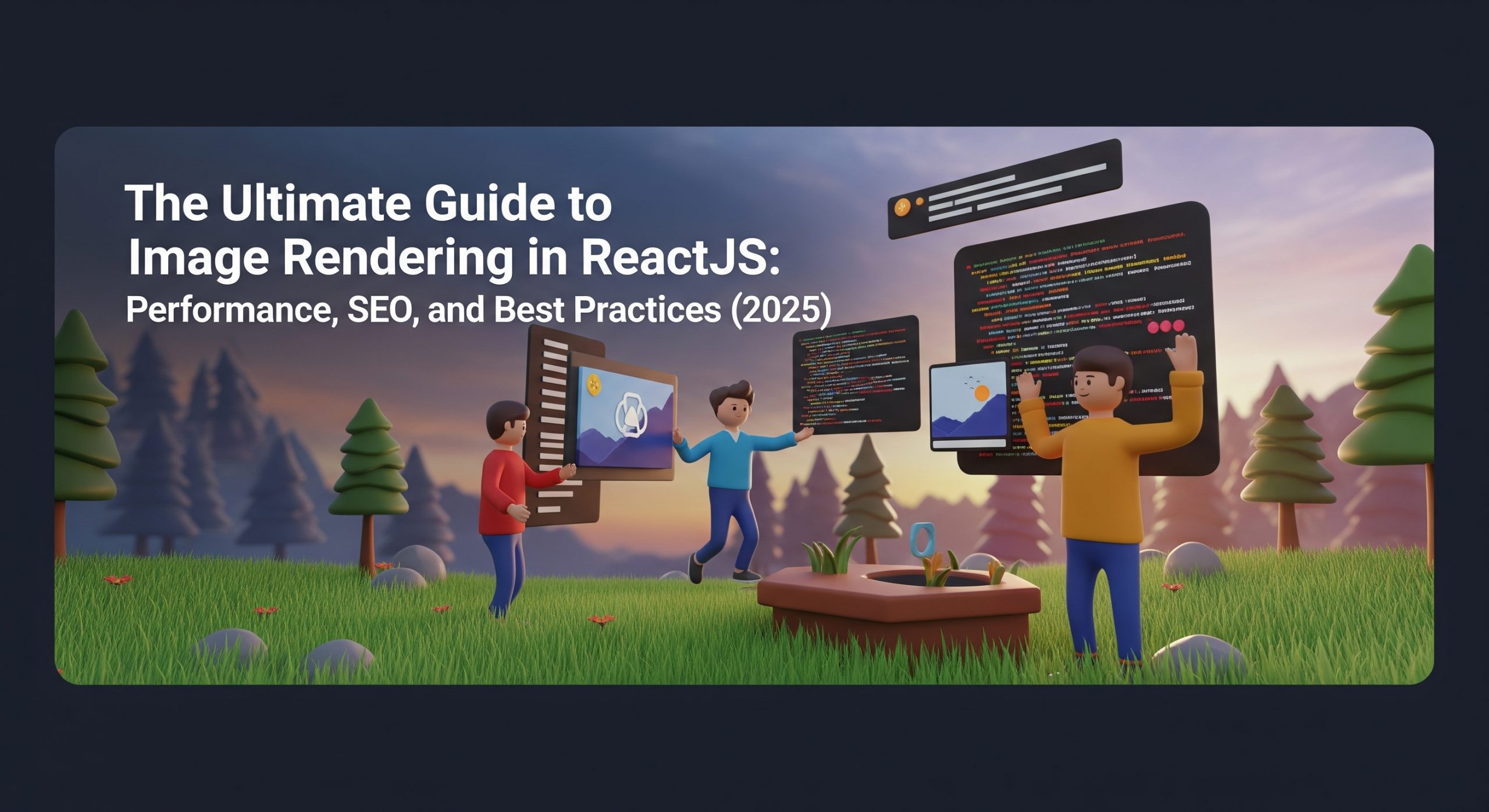
The Ultimate Guide to Image Rendering in ReactJS: Performance, SEO, and Best Practices (2025)
Overview: The Critical Role of Images in Modern Web Development Images are fundamental to web experiences, accounting for approximately 50% of a typical webpage’s total size. How you choose to render these assets significantly impacts user experience, page performance, and search engine rankings. As web technologies evolve, developers have multiple options for implementing images in […]